Hello World: Exploring the Top 12 Programming Languages of 2024
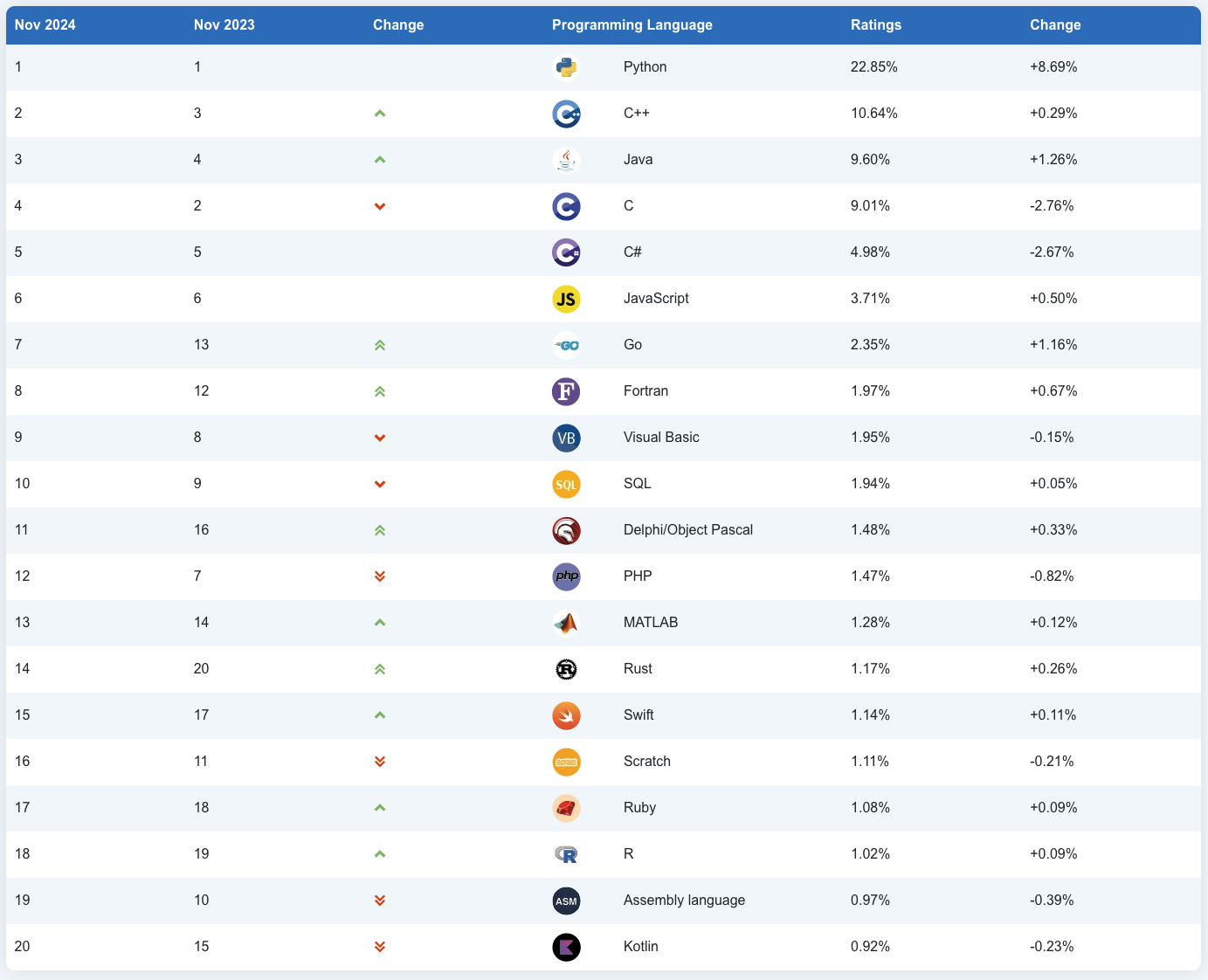
Computer Science 101 tells us a computer’s main job is to transform user input into meaningful output. As my Collin County Community College professor in 1992 put it, “Computers exist to do work for people.”
It’s not the programming language, or even the CPU, doing the thinking. The programming language is simply how a person, called a programmer, tells the computer to complete a task.
Welcome to the official first post of the Code My Way Out (CMWO) blog! I’m thrilled to share my journey as I learn new programming languages and dive deeper into the world of software development. My hope is that this blog becomes a helpful resource as you learn to code your way out of whatever challenges you face.
You Had Me At “Hello, World!”#
To kick off this journey, I wrote a “Hello, World!” program for each of the top 12 programming languages on the TIOBE Index. For those unfamiliar, the TIOBE Index is like a Billboard Top 40 chart for programming languages.
Tweleve “Hello, World!” Function Using TIOBE’s Index of Programming Languages#
#1 - JavaScript#
JavaScript is everywhere. Node.js is a popular server-side runtime for JavaScript, powered by the V8 engine, which also drives the Chrome browser. I don’t know why it took me so long to appreciate this, but I’ve started thinking of the web browser as a local virtual machine—almost like a Docker container—to run your web app.
function hello() {
console.log("Hello, World!");
}
hello();
#2 - Python#
Python is an excellent way to learn programming fundamentals. It has a ton of “wax-on, wax-off” type rules that make you a better programmer. At first, learning those rules feels as exciting as diagramming sentences, but the payoff is worth it! I picked up Python early when it was primarily loved by data scientists. Now it oscillates between #1 and #2 on the TIOBE Index because it’s the go-to language for AI and machine learning.
def hello():
print("Hello, World!")
hello()
#3 - C++#
I probably should have learned C++ first, but I’ve never been much of a linguist. For me, reading about C++ felt like studying Latin when I really needed German, French, or Spanish to get the job done.
#include <iostream>
void hello() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
hello();
return 0;
}
#4 - Java#
Ah, Java—the preferred programming language of neckbeards worldwide! No disrespect to the language or the community, but every time I hear the word “Java,” I get a little whiff of BO. Am I wrong?
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
#5 - C#
C is the classical music of programming languages. Back in the day, learning, programming, and compiling C programs required access to college campuses or corporate computer labs. Now, you can write and compile programs on a $35 Raspberry Pi. That’s progress!
#include <stdio.h>
void hello() {
printf("Hello, World!\n");
}
int main() {
hello();
return 0;
}
#6 - C##
The Microsoft crowd loves C#. It’s like the FrontPage 98 of programming languages.
using System;
class Program {
static void hello() {
Console.WriteLine("Hello, World!");
}
static void Main() {
hello();
}
}
#7 - Go#
Go is more efficient than Python or JavaScript for server-side programming. It’s a great language for building APIs and microservices. I’m excited to learn more about it.
package main
import "fmt"
func hello() {
fmt.Println("Hello, World!")
}
func main() {
hello()
}
#8 - Fortran#
Fortran is the domain of 10x programmers jet-setting on corporate expense accounts to fix legacy code while getting paid mega bucks. You might hate the player, but don’t hate the game. If Fortran is your first language, you’re either in way over your head, retired, or something even worse.
program hello
call hello_world
contains
subroutine hello_world
print *, "Hello, World!"
end subroutine hello_world
end program hello
#9 - Visual Basic#
TIOBE must really need Microsoft as a customer for this “language” to make the list. Can someone explain how VB made the cut?
Module HelloWorld
Sub hello()
Console.WriteLine("Hello, World!")
End Sub
Sub Main()
hello()
End Sub
End Module
#10 - SQL#
SQL is the language of databases. But seriously, how did this make the list? Technically CSS is more popular the SQL, but you don’t see it on the list. My guess SQL programmers spent way more money on “software quality assurance” than the CSS crowd.
SELECT 'Hello, World!';
#11 - Delphi/Object Pascal#
Come on, TIOBE—really? You must need those corporate contracts for Delphi to outrank PHP or even Ruby.
program HelloWorld;
procedure hello;
begin
Writeln('Hello, World!');
end;
begin
hello();
end.
#12 - PHP#
PHP is a scrappy language that is the OG
* “P” (I hear you screaming Perl nerds, but few of us are old enough to remember your glory days) of the LAMP
(Linux, Apache, MySQL, PHP) stack. Yes, there are better, faster, more secure, more maintainable options out there, but that’s not the point. PHP is great not because it’s perfect, but because it’s everywhere. More than any other language, PHP lowered the barrier to entry into professional programming for everyone because of ubiquitous and affordable shared hosting accounts that power most small business websites that still run WordPress, Joomla, and Drupal. PHP may not be your intended, or even desired destination, but, for web developers at least, it will definitely be your original source code. Learn it. Love it. Be confident that there is always a way to get a functional website deployed quickly with PHP. I’d go so far as to say I started learning Python to replace PHP applications that to this day are still humming along in production. That’s because I eventually figured out PHP is actually incredibly awesome. PHP developers deserve way more respect.
* OG
means Original Gangster
<?php
function hello() {
echo "Hello, World!";
}
hello();
?>
Programming Languages Are Like Bands: They Go In and Out of Style#

Check out the long-term trends in the top 20 languages. As a new programmer, your goal isn’t to become a language specialist. Instead, focus on picking the best tool for the job. As a software engineer, your main responsibility is to figure out the tech stack that can be set up, maintained, and supported for the life of your program.
Why is “Hello, World!” the tradional first program for new coders?#
`Hello, World!" is a great teaching tool because it’s a use case everyone undersetands, and there are literally an infinite number of ways to write the program. That beautiful little phrase also reminds us that the heart of every piece of software ever written beats in the human who wrote.